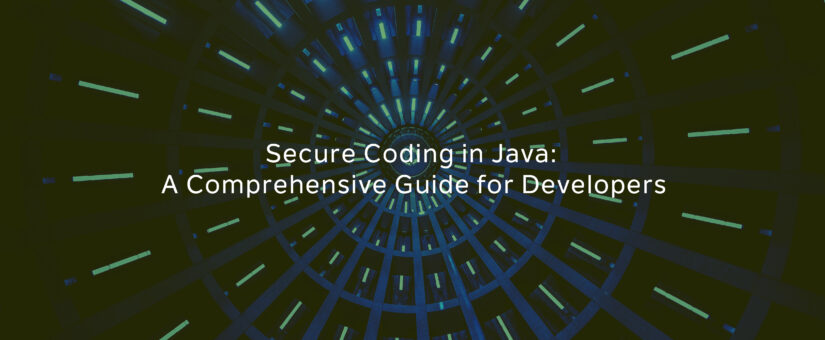
Secure Coding in Java: A Comprehensive Guide for Developers
- Posted by Pierre Malak
- On November 28, 2023
Secure coding is the practice of writing code that is free from security vulnerabilities. This is an essential practice for all software developers, as insecure code can lead to a variety of security breaches and attacks.
In this blog post, we will discuss the importance of secure coding in Java and provide some tips and best practices for writing secure code.
Why is secure coding important?
Secure coding is important for several reasons. First, it can help to protect your software from being exploited by attackers. By writing secure code, you can make it more difficult for attackers to find and exploit vulnerabilities in your software.
Second, secure coding can help to protect your data and the data of your users. By writing secure code, you can help to prevent attackers from accessing sensitive data, such as credit card numbers or personal information.
Third, secure coding can help to protect your reputation. If your software is found to be insecure, it can damage your reputation and lead to a loss of trust from your users.
Software Vulnerabilities Examples:
Integer Overflow:
This security vulnerability happens when an arithmetic operation result is an integer which is too large to be represented within the available variable type. A wrong numerical value will be stored, leading to severe software bugs. To secure your code, check it for integer overflow. This will prevent software bugs and exploits that can be introduced by integer-overflow.
Integer Overflow Fix and Tips
- Use BigInteger for arbitrarily large integers not prone to overflow.
- Validate integer inputs to be within expected ranges.
- Use int or long for loop counters rather than short or byte.
- Methods like Math.addExact() saturate to MAX/MIN_VALUE on overflow
Secure Coding Tips and Best Practices
There are a few things you can do to write secure code in Java. Here are a few tips and best practices:
· Use secure libraries: When using third-party libraries in your Java applications, make sure they are from a trusted source. Avoid using libraries that are known to have security vulnerabilities.
Here are some trusted resources to get code libraries and dependencies from when developing applications:
• Maven Central Repository – The most popular repository for Java libraries. Sources like Apache, Spring, Hibernate publish libraries here.
• Official Vendor Websites – For example, get libraries from Oracle for Java/JDK functionality.
• GitHub – Vetted open-source Java projects published on GitHub by reputable organizations and developers.
• Apache Software Foundation – Source for many stable, production-ready Java libraries under the Apache license.
• Maven Repository – Provides Java libraries under free licenses. Can search based on popularity.
• JCenter – Repository from Bintray providing Java and Android libraries. Used by Gradle & Maven.
• OWASP – Provides recommendations for security focused Java libraries like ESAPI, Cryptacular etc.
The key is sticking to reputable repositories like Maven Central, vendor sites, verified open-source projects on GitHub, Apache/MITRE recommended libraries to avoid including vulnerable or backdoored third party code.
· Validate input: When accepting input from users, always validate it to make sure it is valid and does not contain any malicious code
Example:
- Network requests to suspicious domains or IP addresses.
- Files being written to unusual locations on the filesystem.
- Sensitive data like passwords or keys being sent from the application.
– For Entities validation, use Bean Validation.
//bean Validations public class User { @NotNull private String name; @Size(min=2, max=20) private String username; @Min(18) @Max(60) private int age; @Email private String email; @Pattern(regexp="^\\d{10}$") private String phoneNumber; @NotEmpty private List<String> address; //... }
Validations | Used to |
@NotNull – | Checks field is not null |
@Size – | Checks length of field is between min and max |
@Min – | Checks field is higher than value |
@Max – | Checks field is lower than value |
@Email – | Checks field is valid email format |
@Pattern – | Checks field matches regex pattern |
@NotEmpty – | Checks collection, array, map has elements |
@Past – | Checks date is in the past |
@Future – | Checks date is in the future |
@CreditCardNumber | Checks is valid credit card number |
These bean validation annotations allow declarative validation of bean data in a simple and expressive way.
Validating user inputs is crucial for security, correctness, robustness and preventing bugs. Combining declarative validation annotations, custom Validators, parameter checking, input sanitization, and exception handling provides a robust validation approach in Java.
Handle errors securely: When handling errors in your Java applications, make sure to do so in a secure manner. Avoid disclosing sensitive information to users (ex: Stack trace, UniqueIDs, ..etc).
Use Parameterized SQL Queries: When you use SQL queries try to use prepared statements and parameterized queries instead of concatenating user input
//Safe parameterized query String query = "SELECT * FROM users WHERE id = ?"; PreparedStatement stmt = connection.prepareStatement(query); stmt.setInt(1, userId); //Unsafe concatenated SQL Statement stmt = connection.createStatement("SELECT * FROM users WHERE id = " + userId);
Use secure coding tools: There are a number of tools available that can help you write secure code in Java. These tools can help you find and fix security vulnerabilities in your code.
EX: Static code analysis tool that finds potential bugs in Java code. It can detect a wide range of security vulnerabilities, including buffer overflows, SQL injection, and cross-site scripting.
- FindBugs
- Checkmarx
In summary, writing secure Java code requires a combination of secure coding knowledge, use of protective libraries, input validation, proper error handling, and testing tools. Adopting security best practices while being vigilant against vulnerabilities is key.