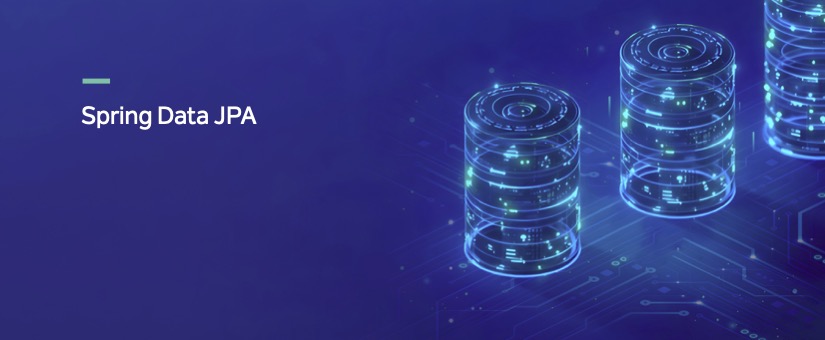
Unlocking the Power of Spring Data JPA
- Posted by Ali Zewil
- On November 24, 2024
In the dynamic landscape of modern software development, efficiency and productivity are paramount. As businesses strive to deliver high-quality applications faster than ever, choosing the right tools and technologies becomes a critical decision. One such tool that has revolutionized data access in Java applications is Spring Data JPA. In this blog post, we’ll delve into the world of Spring Data JPA and explore how it simplifies data access, accelerates development, and empowers your company to build robust applications with ease.
Understanding Spring Data JPA: The Basics
Let’s start by understanding the basics of Spring Data JPA with some code examples.
@Entity @Table(name = "products") public class Product { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; private double price; // Constructors, getters, and setters }
In the code above, we define a JPA entity `Product`, which represents a product in our application. The `@Entity`, `@Table`, and `@Id` annotations are used to specify that this class maps to a database table and its primary key.
Key Benefits of Spring Data JPA
In Spring Data JPA, a repository is an abstraction that provides an interface to interact with a database. It is a part of the Spring Data project, which simplifies the development of data access layers in a Spring application. Repositories in Spring Data JPA are used to perform CRUD (Create, Read, Update, Delete) operations on entities, and they hide the details of how the data is actually stored or retrieved.
- Reduced Boilerplate Code:
Spring Data JPA drastically reduces the boilerplate code required for data access. Let’s take a look at how you can create a repository for the `Product` entity.
public interface ProductRepository extends JpaRepository<Product, Long> { // Custom queries can be added here }
With this simple interface definition, you gain access to a of database operations without writing a single SQL query.
2. Increased Productivity:
The repository then could be used for different components or services.
Below is an example of how it could be used in a service to manipulate your DB:
@Service public class ProductService { private final ProductRepository productRepository; @Autowired public ProductService(ProductRepository productRepository) { this.productRepository = productRepository; } public List<Product> findAllProducts() { return productRepository.findAll(); } public Product saveProduct(Product product) { return productRepository.save(product); } // Other business logic methods }
This code demonstrates how Spring Data JPA allows developers to focus on business logic while automating the underlying database operations.
- Flexibility:
Spring Data JPA provides flexibility in terms of data sources. You can easily switch between different databases or even use multiple data sources within the same application.
- Improved Testing:
Testing is simplified with Spring Data JPA. You can write unit tests for your service classes without involving the database, ensuring comprehensive testing of your application’s business logic.
Getting Started with Spring Data JPA
Getting started with Spring Data JPA is straightforward. You’ll need to include the necessary dependencies in your project’s build configuration and set up a data source.
<
!-- Maven Dependency --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency>
Once you have the dependencies in place, Spring Boot takes care of the configuration, and you can start creating your JPA entities and repositories.
Conclusion
In today’s fast-paced software development landscape, Spring Data JPA stands out as a powerful tool for simplifying data access and streamlining database operations in Java applications. By reducing boilerplate code, improving productivity, and providing a consistent and standardized approach to data access, Spring Data JPA empowers your company to develop robust and feature-rich applications with ease. Incorporating Spring Data JPA into your tech stack not only accelerates development but also enhances code quality, ultimately contributing to the success of your projects.