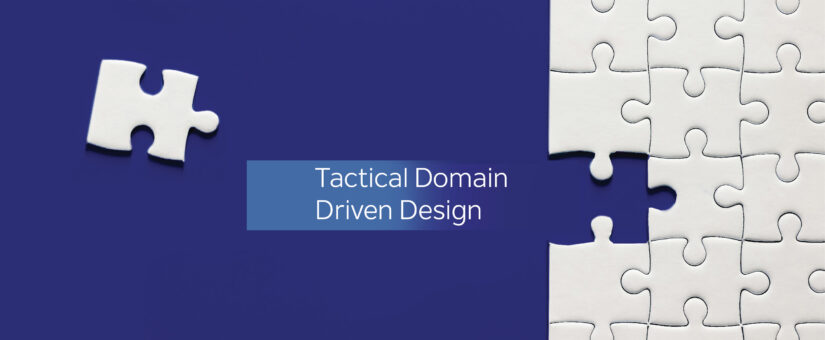
Tactical Domain Driven Design
- Posted by Youssef Refaat
- On June 21, 2023
In the previous article, we talked about strategic design and how it helps us construct bounded contexts, and its relationships. In the next section we will talk about Tactical design.
Tactical Design
Tactical design is a set of tools that helps construct a Domain model for each Bounded context. It helps clarify Business entities and result in a decoupled architecture.
Model Driven Design
Each model can be expressed when translated to a working code as a Service. Its purpose is to serve a single Aggregate. A design that is implemented using a domain model is called Model Driven Design.
Layered Architecture
Creating applications that can handle very complex tasks requires separation of concerns. This is where the concept of Layered Architecture comes in. The basic idea is to structure an application into four conceptual layers.
The “Request Handlers” layer accepts request and passes the needed objects to the right controllers. “Controllers” manage transactions, translates Data Transfer Objects (DTOs) and coordinates application activities. The “Business” layer implements the more domain specific logic. The “Persistence” layer is where the connection to the Data layer is made for persistence purposes. This allows your service to accept requests faster. It is organized, well defined, flexible, reusable, and well maintained
Value Objects
A general-purpose value object is designed to handle complexities and force ubiquitous language.
A well-designed value object has these properties:
- Does not care about uniqueness
- Always immutable
- Rich in domain logic
- Auto Validating
- Has strong equality
- Thread safe
Entities
Entities consist of value objects. They must be uniquely identified by an Id, and we must be able to persist it as a row in a Database. They are typically mutable and must implement some business logic.
Aggregates
Aggregates are a collection of entities and values which come under one transaction boundary. The must have a root entity in which this root entity controls the existence of the aggregate, meaning that if the root entity does not exist, the aggregate does not exist as well. The root entity governs the lifetime of the other entities in the aggregate.
Repositories and Factories
Repositories help you persist aggregates, and that is why there must be one repository per aggregate. Repositories contain the logic behind persisting your aggregates.
Factories help create new aggregates.
Tactical design overview
Building a smart home
To help clarify how DDD design works in real life, let us take an example. We are required to build a full featured smart home. The contractor says that he wants to build a very well-structured smart home and he needs most of the components to be able to communicate with each other. You start implementing right away, and you find out that there are so many questions that needs to be asked. First thing we need to know is what kind of home are we talking about. Is it a one-bedroom apartment, a condo, a standalone apartment, a Villa, or a mansion. What is the kind of components do we need. How many rooms are there, and what type of rooms?
You go back to the contractor and ask him some questions. Two hours into the conversation, you feel overwhelmed by how complex this is getting. So, you go bring your Domain Expert. A domain expert is typically someone who worked on a similar matter before. He has the required business experience that can be useful to speed up this process and understand the complexity well.
Our main Domain is now a Smart Villa. We break down our components until we settle on our bounded contexts. Here is a diagram showing all our BCs
Each component in this diagram can represent a BC. It should have its own value objects, entities, aggregate, etc.
Let us take the lighting for example.
First we create a root entity that can model the Lighting in the right context. The first rule is to never use primitive data types, and instead we composite many value objects that are self-explanatory in terms of language.
Imagine if we use float for brightness for example. This will force our Lighting entity to deal with all changes. If we want to modify the brightness class to add intensity and color, we then only have to modify in the Brightness class as opposed to modifying the whole entity. That is the reason they are called value objects. They are objects that provide value and encapsulate a certain logic that can be described by a ubiquitous language. Any property for an entity that you can describe should be its own class, and then added as an entity variable.
We are now able to see how DDD came to benefit us in designing our classes. Because we could define our domain and sub-domains, we could easily create our domain model which encapsulated several BCs and defined the relationships between them.
We then could create our Ubiquitous language that made us able to name our value objects. These value objects helped us construct a well-structured entity that is consistent and easily understandable because it follows our language standards.
Now let us implement a method that adjusts the brightness.
This is a simple method that takes a float as an input and adjust the brightness accordingly. It first validates that the maximum intensity for the light is not violated, sets the brightness, and then returns the “this” object. We can now notice how the abstraction and intensive use of value objects helped us encapsulate our logic in an organized way.
Summary
Use Domain-Driven Design to collaborate among all project disciplines and clearly understand the business requirements.
Establish a Ubiquitous Language to discuss domain related concepts.
Use Bounded Contexts to break down complex domains into manageable parts.
Implement a Layered Architecture to focus on different aspects of the application.